Mastering MQL5: Practicing Variables and Functions in MT5 - Day 3
Practice working with variables and functions in MetaTrader 5. Learn data types, variable declaration, arithmetic operations, and custom functions to enhance your coding skills in MQL5.
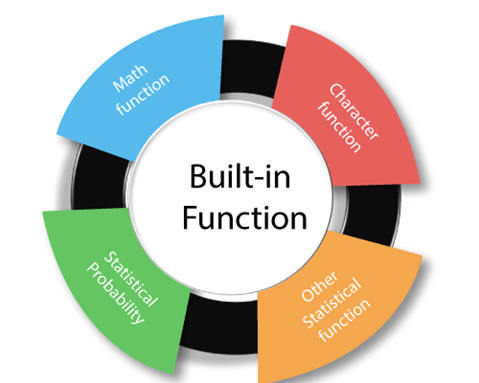

Introduction
Today, we'll focus on hands-on practice with variables and functions in MetaTrader 5 (MT5). Understanding data types, declaring variables, and working with functions are essential skills for building powerful Expert Advisors (EAs). Let's dive into practical examples and elevate your coding proficiency!
Review about Data Types in MQL5
In day 2 off the series, we have learn about it.
- Integer (int)
- Double
- Character (char)
- Boolean (bool)
Leveraging Functions in MT5
Functions are defined with a name, and they can accept input parameters, process them, and optionally return a value. The return value is the output or result of the function's operations.
Example:
// Custom function to calculate the square of a number double Square(double number) { return number * number; } void OnStart() { double num = 5; double squareResult = Square(num); Print("The square of ", num, " is ", squareResult); }
Sorry for the Image not really match the code cause I randomly click refresh the page and all the old content are gone while writing this. Hope you get the idea :D
In summary, functions in MT5 allow us to organize code, pass input parameters, perform operations, and return values. The return value is a critical mechanism to convey the results of a function's calculations or operations back to the main program, enabling efficient communication and facilitating code reuse.
Build-in constant and build-in Functions
In MetaTrader 5 (MT5), there are several built-in constants that provide useful information and values related to trading and the platform.
These constants are predefined and can be directly used in your MQL5 code without the need for explicit declaration.
Example:
double CalculatePositionSize(double riskPercentage, double stopLossPoints) { double riskAmount = GetAccountBalance() * riskPercentage / 100.0; double pointValue = SymbolInfoDouble(_Symbol,SYMBOL_TRADE_TICK_VALUE); Print("_Symbol: ", _Symbol); Print("SYMBOL_TRADE_TICK_VALUE: ", SYMBOL_TRADE_TICK_VALUE); double lotSize = riskAmount / (stopLossPoints * pointValue); return lotSize; } double GetAccountBalance() { return AccountInfoDouble(ACCOUNT_BALANCE); } void OnStart() { double riskPercentage = 2.0; // Double variable to // represent risk percentage double stopLossPoints = 50; Print("Position Size: ", CalculatePositionSize(riskPercentage, stopLossPoints)); }
As you can see, some functions and variables are not defined in our code themself. Such as ACCOUNT_BALANCE, _Symbol, SYMBOL_TRADE_TICK_VALUE or SymbolInfoDouble, ... MT5 cover basic functionality for as by providing this kind of build-in function.
And here is what we got, we can calculate the Position Size base on our risk % and stop-loss.
Here is the list of some of Build-in constant:
_Symbol
: Represents the symbol of the current chart, such as "EURUSD" or "GBPJPY."_Digits
: Indicates the number of decimal places for the current symbol's prices. For example, if_Digits
is 5, the price will be displayed as 1.23456._Point
: Represents the smallest price change for the current symbol. It is equivalent to one pip in most cases._LastError
: Provides the error code of the last operation. It can be used to check if an operation was successful or to handle errors.MODE_BID
: Represents the Bid price of the current symbol. It is the highest price a buyer is willing to pay for the symbol.MODE_ASK
: Represents the Ask price of the current symbol. It is the lowest price a seller is willing to accept for the symbol.OP_BUY
: Indicates a Buy order type.OP_SELL
: Indicates a Sell order type.OP_BUYLIMIT
: Indicates a Buy Limit pending order type.OP_SELLLIMIT
: Indicates a Sell Limit pending order type.OP_BUYSTOP
: Indicates a Buy Stop pending order type.OP_SELLSTOP
: Indicates a Sell Stop pending order type.
They provide valuable information and simplify coding tasks, allowing developers to focus on implementing trading strategies and logic without worrying about manual calculations or data retrieval.
Conclusion
Congratulations on completing Day 3 of our MQL5 programming challenge! You've practiced data types, functions and build-in variable in MT5 with practical examples. In the next day, we will move to Conditional syntax in MQL5. Thanks for reading.